Generate campaign data reports
Moloco Ads APIs for managing campaign data
Moloco provides a comprehensive set of APIs for creating reports with campaign data which you can use to track campaign performance. The following set of APIs are available.
- Create / Read / List / Delete reports. Conceptually, a report is a group of parameters that make up a data report. The parameters include the ad account ID, start and end date, and dimensions (e.g., app, campaign, creative, etc.). Dimensions are the levels at which your campaign data are presented. For example, let's say that you have specified
APP_OR_SITE
as the dimension. In this case, campaign metrics are grouped by each app or site that belongs to the given ad account. - Read report status. Whenever a new report is created, a designated report generation job is created. What the job does is to publish the report to a location where customers can retrieve the actual report files. By calling this API, you can check the status of the job and see where you can download the report files. At this time, you can download the report files in the following formats:
.CSV
and.JSON
.
How Moloco generates data reports
The following is an illustration of how a successful report request looks like from the API layer.
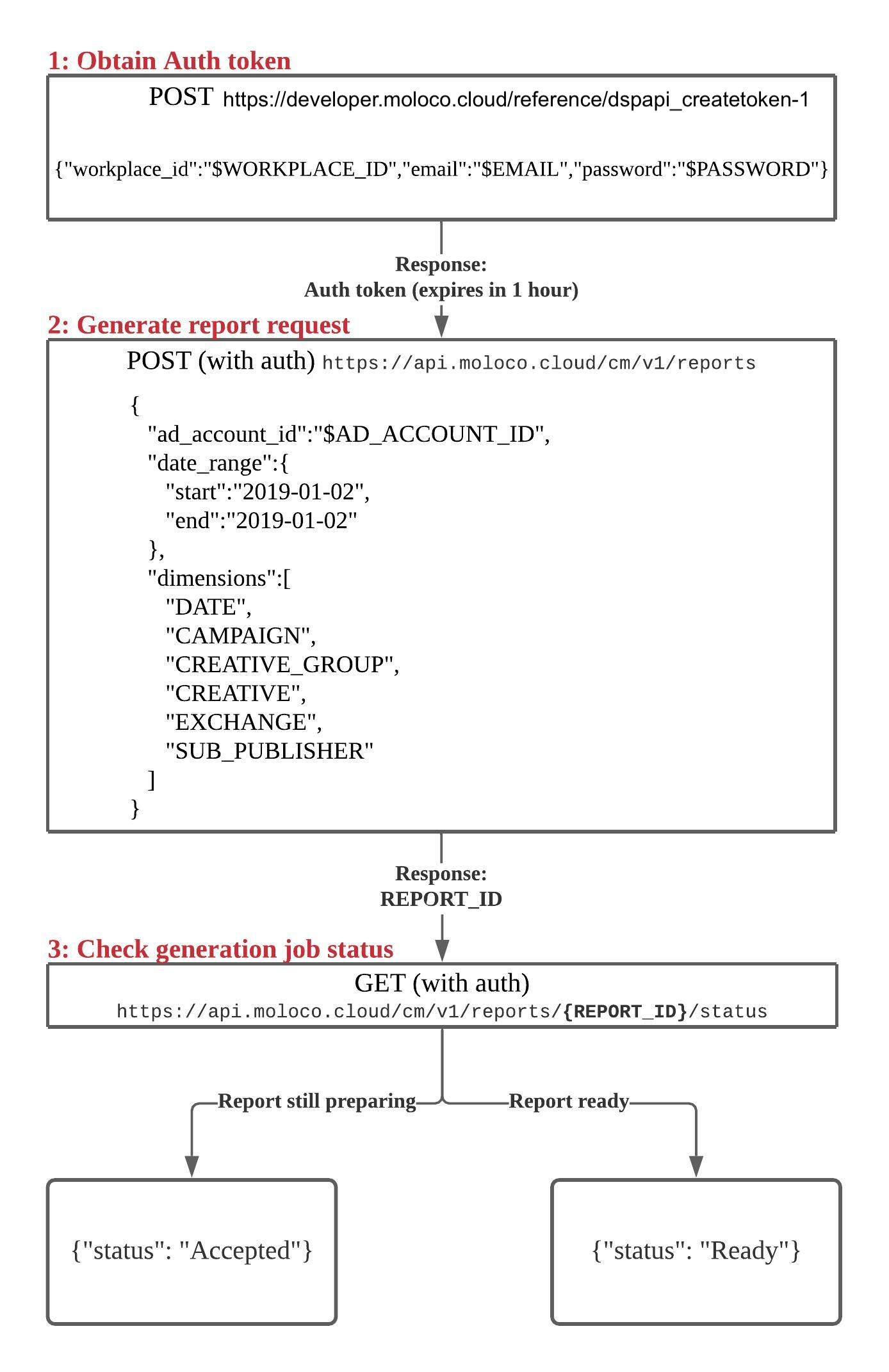
Usage example: Create a Report
To create a report, you must call the Report Creation API with the following parameters: your ad account ID, the date range for campaign data to include in the report, and the dimensions to group campaign data by. At this time, you can specify the following dimensions and optional metrics.
Available dimensions:
DATE
APP_OR_SITE
CAMPAIGN
AD_GROUP
CREATIVE_GROUP
CREATIVE
EXCHANGE
SUB_PUBLISHER
TRAFFIC
SKAN
Optional metrics:
VIDEO_PLAY_PROGRESS
ENGAGED_VIEWS
$ cat report.json
{
"ad_account_id": "$AD_ACCOUNT_ID",
"date_range": {
"start": "2019-01-02",
"end": "2019-01-02"
},
"dimensions": [
"DATE",
"APP_OR_SITE",
"CAMPAIGN",
"AD_GROUP",
"CREATIVE_GROUP",
"CREATIVE",
"EXCHANGE",
"SUB_PUBLISHER",
"TRAFFIC",
"SKAN"
]
}
$ curl -X POST \
-H "Authorization: Bearer $ID_TOKEN" \
-H "Content-Type: application/json" \
-d "@report.json" \ https://api.moloco.cloud/cm/v1/reports
{
"id": "$REPORT_ID",
"href": "https://api.moloco.cloud/cm/v1/reports/$REPORT_ID",
"status": "https://api.moloco.cloud/cm/v1/reports/$REPORT_ID/status"
}
In this example, your report generation parameters are in the report.json
file and delivered to the Report Creation API as an HTTP POST request body. You must replace $AD_ACCOUNT_ID
with your own ad account ID.
In the request response, you can find various information such as (1) your report ID (i.e., $REPORT_ID
), (2) the location where your report creation input is stored (i.e., href
), and (3) the URL where you can check the status of the report generation job (i.e., status
).
In most cases, what you want to do after calling the Report Creation API is to access the URI returned in the status
property to access and download the report files.
Note: You must replace
$ID_TOKEN
with your own access token from Moloco. To learn how to receive an access token, see Get started with Moloco Ads API.
Usage example: Access a report
To access the report you have created, you must call the URI endpoint included in the status
property of the response returned from calling the Report Creation API.
$ curl \
-H "Authorization: Bearer $ID_TOKEN" \
-H "Content-Type: application/json" \
https://api.moloco.cloud/cm/v1/reports/$REPORT_ID/status
{
"status": "READY",
"location_json": "https://$REPORT_STORAGE/$REPORT_ID.json",
"location_csv": "https://$REPORT_STORAGE/$REPORT_ID.csv",
}
In the above example response body, you can see that the report is available in both .json
and .csv
.
After the value of the status
property has updated to READY
as in the example, you can download the report files from the URLs included in location_json
and location_csv
.
Note: The value of
$REPORT_STORAGE
is subject to change, and you must refrain from writing any logic that includes this value.
Schema of a .json
file
.json
fileReport files in .json
format follow the JSON schema below.
{
"$schema": "http://json-schema.org/draft-07/schema#",
"type": "object",
"description": "Contents of a report JSON file consisting of rows.",
"properties": {
"rows": {
"type": "array",
"items": {
"$ref": "#/definitions/Row"
},
"description": "Rows of the report."
}
},
"definitions": {
"Row": {
"type": "object",
"description": "A row in the report table consisting of columns.",
"properties": {
"date": {
"type": "string",
"description": "Date in \"yyyy-mm-dd\" format."
},
"ad_account": {
"$ref": "#/definitions/AdAccountColumn",
"description": "AdAccount column."
},
"app": {
"$ref": "#/definitions/AppColumn",
"description": "App column."
},
"site": {
"$ref": "#/definitions/SiteColumn",
"description": "Site column."
},
"campaign": {
"$ref": "#/definitions/CampaignColumn",
"description": "Campaign column."
},
"ad_group": {
"$ref": "#/definitions/AdGroupColumn",
"description": "Ad-group column."
},
"creative_group": {
"$ref": "#/definitions/CreativeGroupColumn",
"description": "Creative-group column."
},
"creative": {
"$ref": "#/definitions/CreativeColumn",
"description": "Creative column."
},
"exchange": {
"$ref": "#/definitions/ExchangeColumn",
"description": "Exchange column."
},
"sub_publisher": {
"$ref": "#/definitions/SubPublisherColumn",
"description": "Sub-publisher column."
},
"traffic": {
"$ref": "#/definitions/TrafficColumn",
"description": "Traffic column."
},
"metric": {
"$ref": "#/definitions/MetricColumn",
"description": "Metric column."
},
"skan": {
"$ref": "#/definitions/SKANColumn",
"description": "SKAN column."
},
"skan_metric": {
"$ref": "#/definitions/SKANMetricColumn",
"description": "SKAN Metric column."
},
"video_metric": {
"$ref": "#/definitions/VideoMetricColumn",
"description": "Video Metric column."
},
"engaged_view_metric": {
"$ref": "#/definitions/EngagedViewMetricColumn",
"description": "Engaged View Metric Column."
}
}
},
"AdAccountColumn": {
"type": "object",
"description": "AdAccountColumn represents a structured column containing ad-account information.",
"properties": {
"id": {
"type": "string",
"description": "Unique ID of an ad-account."
},
"title": {
"type": "string",
"description": "Descriptive name of an ad-account."
},
"currency": {
"type": "string",
"description": "Contract currency in ISO-4217."
}
}
},
"AppColumn": {
"type": "object",
"description": "AppColumn represents a structured column containing app information.",
"properties": {
"id": {
"type": "string",
"description": "Unique ID of an App."
},
"title": {
"type": "string",
"description": "Descriptive name of an App."
},
"store_id": {
"type": "string",
"description": "ID of an App in the store."
},
"os": {
"type": "string",
"description": "OS type of an App."
}
}
},
"SiteColumn": {
"type": "object",
"description": "SiteColumn represents a structured column containing site information.",
"properties": {
"id": {
"type": "string",
"description": "Unique ID of a Site."
},
"title": {
"type": "string",
"description": "Descriptive name of a Site."
},
"domain": {
"type": "string",
"description": "Domain of a Site."
}
}
},
"CampaignColumn": {
"type": "object",
"description": "CampaignColumn represents a structured column containing campaign information.",
"properties": {
"id": {
"type": "string",
"description": "Unique ID of a campaign."
},
"title": {
"type": "string",
"description": "Descriptive name of a campaign."
},
"country": {
"type": "string",
"description": "Country code in ISO-3166 Alpha-3."
}
}
},
"AdGroupColumn": {
"type": "object",
"description": "AdGroupColumn represents a structured column containing ad-group information.",
"properties": {
"id": {
"type": "string",
"description": "Unique ID of an ad-group."
},
"title": {
"type": "string",
"description": "Descriptive name of an ad-group."
}
}
},
"CreativeGroupColumn": {
"type": "object",
"description": "CreativeGroupColumn represents a structured column containing creative-group information.",
"properties": {
"id": {
"type": "string",
"description": "Unique ID of a creative-group."
},
"title": {
"type": "string",
"description": "Descriptive name of a creative-group."
}
}
},
"CreativeColumn": {
"type": "object",
"description": "CreativeColumn represents a structured column containing creative information.",
"properties": {
"id": {
"type": "string",
"description": "Unique ID of a creative."
},
"title": {
"type": "string",
"description": "Descriptive name of a creative."
},
"type": {
"type": "string",
"description": "Type of a creative."
},
"main_asset_location": {
"type": "string",
"description": "URL of the main asset of a creative."
}
}
},
"ExchangeColumn": {
"type": "object",
"description": "ExchangeColumn represents a structured column containing ad-exchange information.",
"properties": {
"id": {
"type": "string",
"description": "Unique ID of an exchange."
}
}
},
"SubPublisherColumn": {
"type": "object",
"description": "SubPublisherColumn represents a structured column containing sub-publisher information.",
"properties": {
"id": {
"type": "string",
"description": "Unique ID of a sub-publisher."
},
"title": {
"type": "string",
"description": "Descriptive name of a sub-publisher."
}
}
},
"TrafficColumn": {
"type": "object",
"description": "TrafficColumn represents a structured column containing traffic information.",
"properties": {
"is_lat": {
"type": "boolean",
"description": "Flag indicating whether the bid was served with is_lat on or not."
},
"skan_bid": {
"type": "boolean",
"description": "Flag indicating whether the bid of the traffic includes a bid response for SK AdNetwork."
},
"mmp_effective": {
"type": "boolean",
"description": "Flag indicating whether the conversions from the bid can be attributed by MMP."
}
}
},
"MetricColumn": {
"type": "object",
"description": "MetricColumn represents a structured column containing metric numbers.",
"properties": {
"impressions": {
"type": "string",
"format": "int64",
"description": "The number of impressions."
},
"clicks": {
"type": "string",
"format": "int64",
"description": "The number of clicks."
},
"installs": {
"type": "string",
"format": "int64",
"description": "The number of installs."
},
"spend": {
"type": "number",
"format": "double",
"description": "The amount of ad spend in contract currency."
},
"revenue": {
"type": "number",
"format": "double",
"description": "The amount of revenue in contract currency."
}
}
},
"SKANColumn": {
"type": "object",
"description": "SKANColumn represents a structured column containing SK AdNetwork information.",
"properties": {
"conversion_value": {
"type": "string",
"description": "Conversion Value sent by Apple through SK AdNetwork postback."
}
}
},
"SKANMetricColumn": {
"type": "object",
"description": "SKANMetricColumn represents a structured column containing metric numbers related to SK AdNetwork information.",
"properties": {
"conversion_count": {
"type": "string",
"format": "int64",
"description": "The number of counts associated with the corresponding conversion_value."
}
}
},
"VideoMetricColumn": {
"type": "object",
"description": "VideoMetricColumn represents a structured column containing video view progress statistics.",
"properties": {
"video_play_1q": {
"type": "string",
"format": "int64",
"description": "The number of video play for 1st quarter."
},
"video_play_2q": {
"type": "string",
"format": "int64",
"description": "The number of video play for 2nd quarter."
},
"video_play_3q": {
"type": "string",
"format": "int64",
"description": "The number of video play for 3rd quarter."
},
"video_play_4q": {
"type": "string",
"format": "int64",
"description": "The number of video play for 4th quarter."
}
}
},
"EngagedViewMetricColumn": {
"type": "object",
"description": "EngagedViewMetricColumn represents a structured column containing engaged view metric.",
"properties": {
"engaged_views": {
"type": "string",
"format": "int64",
"description": "The number of engaged views."
}
}
}
}
}
Frequently Asked Questions
Why is my report taking so long to load?
The size of the report depends on the date range and dimensions selected in your request. To reduce the time taken to load the report, consider specifying a shorter date range or reducing the number of dimensions to include in your report.
Where can I get $ID_TOKEN
from?
$ID_TOKEN
from?This is a temporary authentication token that has to be generated from a POST request. To learn how to receive an access token, see Get started with Moloco Ads API.
If I would like to get the entire data from the latest date in the report, when would be a good time to run the report?
Campaign data is updated every hour on average, and we recommend waiting until after 3am in your respective timezone to retrieve data from the day before in order to ensure that you have access to all data from the day before.
I would like to pull real-time data for my campaigns. Can I use the Report API to do this?
No, you will have to use the Analytics API instead. See this table for a detailed comparison.
Is there an expiration date for the generated report?
After you have generated a report using the Report API, the report will be available until one month from the date of creation. For example, a report containing data from the first two days of the month of January 2024 that was generated on May 31, 2024 will be available for access until June 31, 2024. After this date, you must create a new report.
Updated 6 months ago