Create and manage your campaign
Moloco Ads APIs for campaign management
We have a comprehensive set of APIs for creating and managing Moloco Ads campaigns. You can create, read, update, delete, and list the following entities.
- AdAccount: This entity represents the advertiser (either an individual or an organization).
- Product: This entity represents the app or website that your campaign advertises.
- TrackingLink: This entity represents the tracking link for your campaign. The tracking link collects data you need to analyze your campaign results.
- Campaign: This entity represents your campaign.
- AdGroup: This entity represents the ad group for your campaign.
- CreativeGroup: This entity represents the creative group, which is a group of creatives used in your campaign.
- Creative: This entity represents the creative asset(s) your campaign uses.
- AudienceTarget: This entity represents a particular group of users your campaign targets.
- CustomerSet: This entity represents a set of users you can use for different purposes.
Entities you need to launch your campaign
In order to launch a new campaign, you need to first create all the entities that make up a campaign. See the entity relationship diagram below for entities you need. If the entity you need has already been created, you can reuse that entity. Otherwise, you must create one.
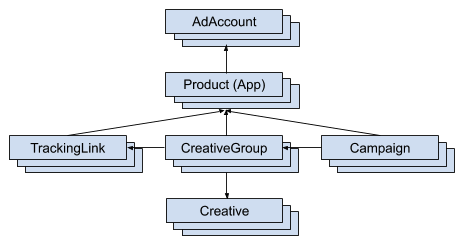
If you are new to Moloco Ads, you should follow the steps below to launch your campaign.
- Create an
ad_account
object that contains information about your AdAccount. - Create a
product
object for the app or website your campaign is promoting. - Create a
tracking_link
object forproduct
. - Create one or more
creative
objects to represent creative assets to be included in your campaign. All creative assets must be uploaded to public storage before you can create acreative
object.
Note: An API that lets you upload a creative asset and create a
creative
object all at once will be made available soon.
- Create one or more
creative_group
objects to represent multiple creatives grouped together. Eachcreative_group
includes one or morecreatives
. You can reuse existingcreative_group
andcreative
objects. - Create a
campaign
object to launch your campaign.
Step-by-step guide to launch a campaign
1. Create an ad_account
object
The first step to creating and launching a campaign is to create an ad_account
object.
The following example shows you how you can use your command-line interface to make an API request to generate an ad_account
object with the request body parameters you have specified as a .json
file. The parameters you have specified for your new ad_account
object are saved to the .json
file and delivered to the Campaign Management API as an HTTP POST request body. For more information about the parameters you can specify, see Create a new ad account.
$ cat ad_account.json
{
"title": "Ad Account For Tutorial",
"description": "Ad Account For Tutorial",
"timezone": "America/Los_Angeles",
"currency": "USD"
}
$ curl -X POST \
-H "Authorization: Bearer $TOKEN" \
-H "Content-Type: application/json" \
-d "@ad_account.json" \
"https://api.moloco.cloud/cm/v1/ad-accounts"
{
"ad_account": {
"id": "$AD_ACCOUNT_ID",
"title": "Ad Account For Tutorial",
"description": "Ad Account For Tutorial",
"timezone": "America/Los_Angeles",
"currency": "USD",
"created_at": "2020-07-01T00:00:17.317689Z",
"updated_at": "2020-07-01T00:00:17.317689Z"
}
}
As in the example, the response includes the Moloco-issued ID for the AdAccount entity. Your AdAccount ID is the value of the id
property in the ad_account
object. For more information about each of the parameters included in the response, see Create a new ad account.
Note: To make an API request, you need a Moloco-issued token to include in the request body. To learn how to receive a token for authentication, see Get Started with Moloco Ads API.
2. Create a product
object
After you have a created an ad_account
object, you must create a product
object. You need to include in the request body the AdAccount ID that was created in the previous step.
The following example shows you how you can use your command-line interface to make an API request to generate a product
object with the request body parameters you have specified as a .json
file. The parameters you have specified for your new product
object are saved to the .json
file and delivered to the Campaign Management API as an HTTP POST request body. For more information about the parameters you can specify, see Create a new product.
$ cat product.json
{
"title": "Tutorial App",
"advertiser_domain": "molocoads.com",
"device_os": "ANDROID",
"type": "APP",
"app": {
"bundle_id": "com.moloco.ads",
"category": "IAB9-1",
"rating": 5,
"app_store_url": "https://play.google.com/store/apps/details?id=com.moloco.ads",
"postback_integration": {
"mmp": "TRACKFNT",
"bundle_id": "com.moloco.ads"
}
}
}
$ curl -X POST \
-H "Authorization: Bearer $TOKEN" \
-H "Content-Type: application/json" \
-d "@product.json" \
"https://api.moloco.cloud/cm/v1/products?ad_account_id=$AD_ACCOUNT_ID"
{
"product": {
"ad_account_id": "$AD_ACCOUNT_ID",
"id": "$PRODUCT_ID",
"title": "Tutorial App",
"advertiser_domain": "molocoads.com",
"device_os": "ANDROID",
"type": "APP",
"app": {
"bundle_id": "com.moloco.ads",
"category": "IAB9-1",
"rating": 5.0,
"app_store_url": "https://play.google.com/store/apps/details?id=com.moloco.ads",
"tracking_company": "ADJUST",
"tracking_bundle_id": "com.moloco.ads",
"postback_integration": {
"mmp": "TRACKFNT",
"bundle_id": "com.moloco.ads"
},
"mmp_integration": {
"id": "mAZE5cU0tZl1uIA5",
"mmp_bundle_id": "com.moloco.ads",
"mmp": "ADJUST",
"status": "UNDER_REVIEW",
"updated_at": "2020-07-01T15:29:45.457575Z"
}
},
"created_at": "2020-07-01T15:29:45.732976Z",
"updated_at": "2020-07-01T15:29:45.732976Z"
}
}
As in the example, the response includes the Moloco-issued ID for the Product entity. Your Product ID is the value of the id
property in the product
object. For more information about each of the parameters included in the response, see Create a new Product.
3. Create a tracking_link
object
You must create a tracking_link
object to track your campaign's performance. The tracking_link
object includes the name of tracking company (e.g., Adjust, Airbridge, AppsFlyer, etc.), click-through (CT) link, and view-through (VT) link. The CT link notifies the tracking company of any click events, and the VT link notifies them of any impression events. To create a tracking_link
object, you need to enter the name of tracking company your app is using, the CT link, and an optional VT link issued by the tracking company.
The following example shows you how you can use your command-line interface to make an API request to generate a tracking_link
object with the request body parameters you have specified as a .json
file. The parameters you have specified for your new tracking_link
object are saved to the .json
file and delivered to the Campaign Management API as an HTTP POST request body. For more information about the parameters you can specify, see Create a new tracking link.
$ cat tracking_link.json
{
"title": "Tutorial App Tracking Link",
"description": "Tutorial App Tracking Link",
"device_os": "ANDROID",
"click_through_link": {
"url": "https://tracker-us.adsmoloco.com/com.trackfnt.adNetworkTest?advertising_id=%{a_idfa}&mt_ad=%{creative_filename}&mt_ad_id=%{creative_id}&mt_ad_type=%{creative_type}&mt_click_lookback=7d&mt_cost_currency=%{cost_currency}&mt_cost_model=%{cost_type}&mt_cost_value=%{cost_amount}&mt_siteid=%{raw_app_bundle}&mt_sub4=%{source}&c=%{campaign}&clickid=%{bid_id}&pid=moloco_int"
},
"view_through_link": {
"url": "https://tracker-us.adsmoloco.com/com.trackfnt.adNetworkTest?advertising_id=%{a_idfa}&mt_ad=%{creative_filename}&mt_ad_id=%{creative_id}&mt_ad_type=%{creative_type}&mt_cost_currency=%{cost_currency}&mt_cost_model=%{cost_type}&mt_cost_value=%{cost_amount}&mt_siteid=%{raw_app_bundle}&mt_sub4=%{source}&mt_viewthrough_lookback=1d&c=%{campaign}&clickid=%{bid_id}&pid=moloco_int"
},
"tracking_company": "TRACKFNT"
}
$ curl -X POST \
-H "Authorization: Bearer $TOKEN" \
-H "Content-Type: application/json" \
-d "@tracking_link.json" \
"https://api.moloco.cloud/cm/v1/tracking-links?ad_account_id=$AD_ACCOUNT_ID&product_id=$PRODUCT_ID"
{
"tracking_link": {
"id": "$TRACKING_LINK_ID",
"ad_account_id": "$AD_ACCOUNT_ID",
"product_id": "$PRODUCT_ID",
"title": "Tutorial App Tracking Link",
"description": "Tutorial App Tracking Link",
"device_os": "ANDROID",
"click_through_link": {
"url": "https://tracker-us.adsmoloco.com/com.trackfnt.adNetworkTest?advertising_id=%{a_idfa}&ml_ad=%{creative_filename}&ml_ad_id=%{creative_id}&ml_ad_type=%{creative_type}&ml_click_lookback=7d&ml_cost_currency=%{cost_currency}&ml_cost_model=%{cost_type}&ml_cost_value=%{cost_amount}&ml_siteid=%{raw_app_bundle}&ml_sub4=%{source}&c=%{campaign}&clickid=%{bid_id}&pid=moloco_int",
"status": "UNVERIFIED",
"unverified_status_data": {
"stored_at": "2020-07-01T09:50:37Z"
}
},
"view_through_link": {
"url": "https://tracker-us.adsmoloco.com/com.trackfnt.adNetworkTest?advertising_id=%{a_idfa}&ml_ad=%{creative_filename}&ml_ad_id=%{creative_id}&ml_ad_type=%{creative_type}&ml_cost_currency=%{cost_currency}&ml_cost_model=%{cost_type}&ml_cost_value=%{cost_amount}&ml_siteid=%{raw_app_bundle}&ml_sub4=%{source}&ml_viewthrough_lookback=1d&c=%{campaign}&clickid=%{bid_id}&pid=moloco_int",
"status": "UNVERIFIED",
"unverified_status_data": {
"stored_at": "2020-07-01T09:50:37Z"
}
},
"tracking_company": "TRACKFNT",
"created_at": "2020-07-01T09:50:37.924722Z",
"updated_at": "2020-07-01T09:50:37.924722Z"
}
}
As in the example, the response includes the Moloco-issued TrackingLink ID that you need when creating a creative_group
object. Your TrackingLink ID is the value of the id
property in the tracking_link
object. For more information about each of the parameters included in the response, see Create a new tracking link.
4. Upload a creative asset and create a creative
object
For each of your campaigns, you must create at least one creative
object to add to a creative_group
object. Every campaign must have at least one CreativeGroup entity, and each CreativeGroup entity can include multiple Creative entities.
4-1. Upload a creative asset to storage (for those using Moloco Ads API v1.6 or earlier)
Keep in mind:
If you are using Moloco Ads API v1.6 or earlier, you must proceed with the following to upload your creative asset(s).
To create a creative
object for your creative asset, you must first upload the creative asset file. The following limitations apply to each file.
- Image: Up to 500KB
- Video: Up to 10MB
- GIF: Up to 1MB
Note: If using Moloco Ads API v1.5 or later, your video files are automatically transcoded to optimize performance. An error message is returned in the event that the file exceeds the size limit after it has been transcoded. For more information, see the release note.
To upload a creative asset, you must do the following.
- Make a POST API request to get a Moloco-issued URL for uploading your creative asset.
- Make a POST API request to initiate a resumable upload session for the creative asset.
- Make a PUT API request with the Moloco-issued URL to upload the creative asset to Google Cloud Storage.
The following example shows you how you can use your command-line interface to make API requests to get a Moloco-issued URL for your creative asset and upload the creative asset to storage. To learn more about the parameters you can specify, see Create a new asset upload session.
The first step is making a POST API request with the required parameters as in the following example.
$ cat asset.json
{
"asset_kind": "CREATIVE",
"mime_type": "image/jpeg",
"file_name": "test_image.jpg"
}
$ curl -X POST \
-H "Authorization: Bearer $TOKEN" \
-H "Content-Type: application/json" \
-d "@asset.json" \
"https://api.moloco.cloud/cm/v1/creative-assets?ad_account_id=$AD_ACCOUNT_ID"
{
"asset_url": "$CREATIVE_ASSET_URL",
"content_upload_url": "$UPLOAD_URL"
}
As in the example, the response includes the asset URL and upload URL. The asset URL, which is the value of the asset_url
property, is the public address where your creative asset is made available after it has been uploaded to Google Cloud Storage. The upload URL, which is the value of the content_upload_url
property, is for uploading the creative asset file to Google Cloud Storage.
You must make another POST API request with the upload URL to start a resumable upload session. The upload URL points to the URL for resumable upload. You can initiate a resumable upload session as follows.
$ curl -v -X POST \
-H "x-goog-resumable: start" \
-H "Content-Type: image/jpeg" \
"$UPLOAD_URL" 2>&1 \
| grep "x-guploader-uploadid"
< x-guploader-uploadid: $UPLOAD_ID
Finally, you must make a PUT API request with the upload URL and upload ID to upload the creative asset file to Google Cloud Storage. You must include in the request $ASSET_PATH
, which is the location the file is saved in (e.g., my-dir/file.jpg
). Be sure to append the upload ID returned in the previous POST API request to the upload URL when making the request as in the following example.
$ curl -X PUT \
--data-binary "@$ASSET_PATH" \
-H "Content-Type: image/jpeg" \
"$UPLOAD_URL&upload_id=$UPLOAD_ID"
Your creative asset has been uploaded to Google Cloud Storage, and is available at the URL specified for asset_url
, which you need for creating a new creative
object.
4-1. Upload a creative asset to storage (for those using Moloco Ads API v1.7 or later)
Keep in mind:
If you are using Moloco Ads API v1.7 or later, you must proceed with the following to upload your creative asset(s).
To create a creative
object for your creative asset, you must first upload the creative asset file. The following limitations apply to the types of assets you can specify as the value of the asset_kind
property.
Asset type | Maximum file size |
---|---|
CREATIVE, ASSET_DYNAMIC_CREATIVE | 200MB |
CSV | 4GB |
FB_PLAYABLE_AD | 5MB |
Note: If using Moloco Ads API v1.5 or later, your video files are automatically transcoded to optimize performance. An error message is returned in the event that the file exceeds the size limit after it has been transcoded. For more information, see the release note.
To upload a creative asset, you must do the following.
- Make a POST API request to get a session URL for uploading your creative asset to Google Cloud Storage.
- Make a PUT API request with the session URL to upload the creative asset to Google Cloud Storage.
The following example shows you how you can use your command-line interface to make API requests to get a session URL for your creative asset and upload the creative asset to storage. To learn more about the parameters you can specify, see Create a new asset upload session.
The first step is making a POST API request with the required parameters as in the following example.
$ cat asset.json
{
"asset_kind": "CREATIVE",
"mime_type": "image/jpeg"
}
$ curl -X POST \
-H "Authorization: Bearer $TOKEN" \
-H "Content-Type: application/json" \
-d "@asset.json" \
"https://api.moloco.cloud/cm/v1/creative-assets?ad_account_id=$AD_ACCOUNT_ID"
{
"asset_url": "$CREATIVE_ASSET_URL",
"content_upload_url": "$UPLOAD_URL"
}
As in the example, the response includes the asset URL and upload URL. The asset URL, which is the value of the asset_url
property, is the public address where your creative asset is made available after it has been uploaded to Google Cloud Storage. The upload URL, which is the value of the content_upload_url
property, is for uploading the creative asset file to Google Cloud Storage.
You must make a PUT API request with the upload URL and upload ID to upload the creative asset file to Google Cloud Storage. You must include in the request $ASSET_PATH
, which is the location the file is saved in (e.g., my-dir/file.jpg
).
$ curl -X PUT \
--data-binary "@$ASSET_PATH" \
-H "Content-Type: image/jpeg" \
$UPLOAD_URL
Your creative asset has been uploaded to Google Cloud Storage, and is available at the URL specified for asset_url
, which you need for creating a new creative
object.
4-2. Create a creative
object
After your creative asset has been uploaded to Google Cloud Storage and assigned a public URL, you must create a creative
object.
The following example shows you how you can use your command-line interface to make an API request to generate a creative
object with the request body parameters you have specified as a .json
file. The parameters you have specified for your new creative
object are saved to the .json
file and delivered to the Campaign Management API as an HTTP POST request body. For more information about the parameters you can specify, see Create a new creative.
$ cat creative.json
{
"title": "Test Image Creative",
"type": "IMAGE",
"original_filename": "test_image.jpg",
"size_in_bytes": 94994,
"image": {
"image_url": "$CREATIVE_ASSET_URL",
"width": 640,
"height": 960,
"size_in_bytes": 94994
}
}
$ curl -X POST \
-H "Authorization: Bearer $TOKEN" \
-H "Content-Type: application/json" \
-d "@creative.json" \
"https://api.moloco.cloud/cm/v1/creatives?ad_account_id=$AD_ACCOUNT_ID&product_id=$PRODUCT_ID"
{
"creative": {
"id": "$CREATIVE_ID",
"ad_account_id": "$AD_ACCOUNT_ID",
"title": "Test Image Creative",
"enabling_state": "ENABLED",
"ad_account_info": {
"id": "$AD_ACCOUNT_ID",
"title": "Ad Account For Tutorial",
"description": "Ad Account For Tutorial"
},
"type": "IMAGE",
"original_filename": "test_image.jpg",
"size_in_bytes": 94994,
"image": {
"image_url": "$CREATIVE_ASSET_URL",
"width": 640,
"height": 960,
"size_in_bytes": 94994
},
"created_at": "2020-07-01T14:54:00.526009Z",
"updated_at": "2020-07-01T14:54:00.526009Z"
}
}
As in the example, the response includes the Moloco-issued Creative ID you need when creating a creative_group
object. The Creative ID is the value of the id
property in the creative
object. For more information about each of the parameters included in the response, see Create a new creative.
5. Create a creative_group
object
You can group Creative entities with similar characteristics under a CreativeGroup entity to make it easier to add them to the campaigns of your choice. To create a creative_group
object, you must have created one or more creative
objects as well as a tracking_link
object.
The following example shows you how you can use your command-line interface to make an API request to generate a creative_group
object with the request body parameters you have specified as a .json
file. The parameters you have specified for your new creative_group
object are saved to the .json
file and delivered to the Campaign Management API as an HTTP POST request body. For more information about the parameters you can specify, see Create a new creative group.
$ cat creative_group.json
{
"ad_account_id": "$AD_ACCOUNT_ID",
"product_id": "$PRODUCT_ID",
"title": "Test CreativeGroup",
"description": "Test CreativeGroup",
"enabling_state": "ENABLED",
"status": "APPROVED",
"tracking_link_id": "$TRACKING_LINK_ID",
"creative_ids": [
"$CREATIVE_ID"
]
}
$ curl -X POST \
-H "Authorization: Bearer $TOKEN" \
-H "Content-Type: application/json" \
-d "@creative_group.json" \
"https://api.moloco.cloud/cm/v1/creative-groups?ad_account_id=$AD_ACCOUNT_ID&product_id=$PRODUCT_ID"
{
"creative_group": {
"id": "tla69ad6dz2JAUAY",
"ad_account_id": "$AD_ACCOUNT_ID",
"product_id": "$PRODUCT_ID",
"title": "Test CreativeGroup",
"description": "Test CreativeGroup",
"enabling_state": "ENABLED",
"creative_ids": [
"$CREATIVE_ID"
],
"status": "APPROVED",
"action_history": [
{
"id": "05fb3aa5-44b9-4943-77c4-90f5f15a31c7",
"action_type": "CREATE",
"created_at": "2020-07-01T15:21:05.797660752Z"
}
],
"tracking_link_id": "$TRACKING_LINK_ID",
"audience": {},
"created_at": "2020-07-01T15:21:05.909989Z",
"updated_at": "2020-07-01T15:21:05.909989Z"
},
"creatives": [
{
"id": "$CREATIVE_ID",
"ad_account_id": "$AD_ACCOUNT_ID",
"title": "Test Image Creative",
"enabling_state": "ENABLED",
"ad_account_info": {
"id": "$AD_ACCOUNT_ID",
"title": "Ad Account For Tutorial",
"description": "Ad Account For Tutorial"
},
"type": "IMAGE",
"original_filename": "test_image.jpg",
"size_in_bytes": 94994,
"image": {
"image_url": "$CREATIVE_ASSET_URL",
"width": 640,
"height": 960,
"size_in_bytes": 94994
},
"created_at": "2020-07-01T14:54:00.526009Z",
"updated_at": "2020-07-01T14:54:00.526009Z"
}
],
"tracking_link": {
"id": "$TRACKING_LINK_ID",
"ad_account_id": "$AD_ACCOUNT_ID",
"product_id": "$PRODUCT_ID",
"title": "Tutorial App Tracking Link",
"description": "Tutorial App Tracking Link",
"device_os": "ANDROID",
"click_through_link": {
"url": "https://tracker-us.adsmoloco.com/com.trackfnt.adNetworkTest?advertising_id=%{a_idfa}&ml_ad=%{creative_filename}&ml_ad_id=%{creative_id}&ml_ad_type=%{creative_type}&ml_click_lookback=7d&ml_cost_currency=%{cost_currency}&ml_cost_model=%{cost_type}&ml_cost_value=%{cost_amount}&ml_siteid=%{raw_app_bundle}&ml_sub4=%{source}&c=%{campaign}&clickid=%{bid_id}&pid=moloco_int",
"status": "UNVERIFIED",
"unverified_status_data": {
"stored_at": "2020-07-01T09:50:37Z"
}
},
"view_through_link": {
"url": "https://tracker-us.adsmoloco.com/com.trackfnt.adNetworkTest?advertising_id=%{a_idfa}&ml_ad=%{creative_filename}&ml_ad_id=%{creative_id}&ml_ad_type=%{creative_type}&ml_cost_currency=%{cost_currency}&ml_cost_model=%{cost_type}&ml_cost_value=%{cost_amount}&ml_siteid=%{raw_app_bundle}&ml_sub4=%{source}&ml_viewthrough_lookback=1d&c=%{campaign}&clickid=%{bid_id}&pid=moloco_int",
"status": "UNVERIFIED",
"unverified_status_data": {
"stored_at": "2020-07-01T09:50:37Z"
}
},
"tracking_company": "TRACKFNT",
"created_at": "2020-07-01T09:50:37.924722Z",
"updated_at": "2020-07-01T09:50:37.924722Z"
}
}
As in the example, the response includes the Moloco-issued CreativeGroup ID. The CreativeGroup ID is the value of the id
property in the creative_group
object. For more information about each of the parameters included in the response, see Create a new creative group.
6. Create a campaign
object
Finally, you must create a campaign
object using information from the previous steps.
The following example shows you how you can use your command-line interface to make an API request to generate a campaign
object with the request body parameters you have specified as a .json
file. The parameters you have specified for your new campaign
object are saved to the .json
file and delivered to the Campaign Management API as an HTTP POST request body. For more information about the parameters you can specify, see Create a new campaign.
$ cat campaign.json
{
"campaign": {
"ad_account_id": "$AD_ACCOUNT_ID",
"product_id": "$PRODUCT_ID",
"title": "Test Campaign",
"description": "Test Campaign",
"enabling_state": "ENABLED",
"type": "APP_USER_ACQUISITION",
"device_os": "ANDROID",
"country": "KOR",
"currency": "USD",
"schedule": {
"start": "2020-07-01T07:00:00Z"
},
"budget_schedule": {
"daily_budget": {
"daily_budget": {
"currency": "USD",
"amount_micros": "1000000"
}
}
},
"tracking_company": "TRACKFNT",
"goal": {
"type": "OPTIMIZE_CPI_FOR_APP_UA",
"optimize_app_installs": {
"mode": "TARGET_CPI_CENTRIC",
"target_cpi": {
"currency": "USD",
"amount_micros": "1000000"
}
}
}
},
"ad_group": {
"title": "Test AdGroup",
"enabling_state": "ENABLED",
"audience": {},
"capper": {
"imp_interval": {
"amount": "12",
"unit": "HOUR"
}
},
"creative_group_ids": [
"$CREATIVE_GROUP_ID"
]
}
}
$ curl -X POST \
-H "Authorization: Bearer $TOKEN" \
-H "Content-Type: application/json" \
-d "@campaign.json" \
"https://api.moloco.cloud/cm/v1/campaigns?ad_account_id=$AD_ACCOUNT_ID&product_id=$PRODUCT_ID"
{
"campaign": {
"id": "$CAMPAIGN_ID",
"ad_account_id": "$AD_ACCOUNT_ID",
"product_id": "$PRODUCT_ID",
"title": "Test Campaign",
"description": "Test Campaign",
"enabling_state": "ENABLED",
"type": "APP_USER_ACQUISITION",
"device_os": "ANDROID",
"state": "SUBMITTED",
"country": "KOR",
"currency": "USD",
"schedule": {
"start": "2020-07-01T07:00:00Z"
},
"budget_schedule": {
"daily_budget": {
"daily_budget": {
"currency": "USD",
"amount_micros": "1000000"
}
}
},
"audience": {
"shared_audience_target_ids": [],
"targeting_condition": {
"allowed_exchanges": [
"ADX",
"MOPUB",
"UNITY"
]
}
},
"tracking_company": "TRACKFNT",
"goal": {
"type": "OPTIMIZE_APP_INSTALLS",
"optimize_app_installs": {
"mode": "TARGET_CPI_CENTRIC",
"target_cpi": {
"currency": "USD",
"amount_micros": "1000000"
}
}
},
"created_at": "2020-07-01T15:34:43.323228Z",
"updated_at": "2020-07-01T15:34:43.323228Z"
}
}
As in the example, the response includes the Campaign ID, which is the value of the id
property of the campaign
object. For more information about each of the parameters included in the response, see Create a new campaign.
After a campaign
object has been created, the state
property indicating campaign status is set to SUBMITTED
by default. When the ML model for the campaign is ready, campaign status is updated to either READY
or UPCOMING
depending on the value of the enabling_state
property. Campaign status is updated to READY
when the value of the enabling_state
property is set to DISABLED
. Campaign status is updated to UPCOMING
when the value of the enabling_state
property is set to ENABLED
.
Note: It usually takes about 2 hours for the ML model for your campaign to finish training.
On the date and time campaign is scheduled to start running, campaign status is updated to PAUSED
when the value of the enabling_state
property is set to DISABLED
or ACTIVE
when the value of the enabling_state
property is set to ENABLED
. When campaign status is ACTIVE
, the creatives added to the campaign are live on Moloco Ads, and the campaign can start bidding. To learn how to track campaign performance, see Report API and Log API.
In the event that the campaign has a specified end date, campaign status is updated to COMPLETED
on the date and time campaign is scheduled to end.
Note: When a campaign has been deleted, the campaign status is updated to
COMPLETED
. Campaigns that have been inPAUSED
status for more than 180 days are converted toCOMPLETED
and subsequently deleted in compliance with Moloco's data access policy.
Usage example: Create a customer_set
object
customer_set
object1. Create a customer_set
object
A CustomerSet entity represents a set of customer IDFAs. You can use this entity to include in or exclude from targeting a specific group of customers. To create a customer_set
object, you must upload a .csv
file with customer IDFAs to Google Cloud Storage.
1-1. Upload a CustomerSet asset to Google Cloud Storage using Moloco Ads API
To upload a CustomerSet asset to Google Cloud Storage, you must do the following.
- Make a POST API request to get a Moloco-issued URL for uploading your CustomerSet asset.
- Make a POST API request to initiate a resumable upload session for the CustomerSet asset.
- Make a PUT API request with the Moloco-issued URL to upload the CustomerSet asset.
The following example shows you how you can use your command-line interface to make API requests to get a Moloco-issued URL for your CustomerSet asset and upload the CustomerSet asset to storage. To learn more about the parameters you can specify, see Create a new asset upload session.
You must first make a POST API request with the required parameters to get a URL for uploading your CustomerSet asset as in the following example.
$ cat asset.json
{
"asset_kind": "CSV",
"mime_type": "text/csv",
"file_name": "test_customer_set.csv"
}
$ curl -X POST \
-H "Authorization: Bearer $TOKEN" \
-H "Content-Type: application/json" \
-d "@asset.json" \
"https://api.moloco.cloud/cm/v1/creative-assets?ad_account_id=$AD_ACCOUNT_ID"
{
"asset_url": "$CUSTOMER_SET_ASSET_URL",
"content_upload_url": "$UPLOAD_URL"
}
As in the example, the response includes the asset URL and upload URL. The asset URL, which is the value of the asset_url
property, is the public address where your CustomerSet asset is made available after it has been uploaded to Google Cloud Storage. The upload URL, which is the value of the content_upload_url
property, is for uploading the CustomerSet asset file to Google Cloud Storage.
You must make another POST API request with the upload URL to start a resumable upload session. The upload URL points to the URL for resumable upload. You can initiate a resumable upload session as follows.
$ curl -v -X POST \
-H "x-goog-resumable: start" \
-H "Content-Type: text/csv" \
"$UPLOAD_URL" 2>&1 \
| grep "x-guploader-uploadid"
< x-guploader-uploadid: $UPLOAD_ID
Finally, you must make a PUT API request with the upload URL and upload ID to upload the CustomerSet asset file to Google Cloud Storage. You must include in the request $ASSET_PATH
, which is the location the file is saved in (e.g., my-dir/file.jpg). Be sure to append the upload ID returned in the previous POST API request to the upload URL when making the request as in the following example.
$ curl -i -X PUT \
--data-binary "@$ASSET_PATH" \
-H "Content-Type: text/csv" \
-H "Connection: keep-alive" \
"$UPLOAD_URL&upload_id=$UPLOAD_ID"
When you see that HTTP 200 or 201 is returned as the response, the asset file has been successfully uploaded to storage. You can access the file using the asset URL, which you need when creating a customer_set
object.
Tip: Check the integrity of the uploaded file
To check whether the file you have uploaded has been tampered with, you can make a PUT API request as in the following example.
$ curl -i -X PUT \ -H "Content-Length: 0" \ -H "Content-Range: bytes */*" \ "$UPLOAD_URL&upload_id=$UPLOAD_ID" 2>&1 \ | grep "x-goog-hash\|HTTP\|x-goog-stored-content-encoding" HTTP/2 200 x-goog-hash: crc32c=$GOOG_CRC32C x-goog-hash: md5=$GOOG_HASH x-goog-stored-content-encoding: identity
If your local machine has
gsutil
installed, you can verify file integrity by comparing$GOOG_HASH
and$FILE_HASH
as in the following example. If the two values are the same, your file is safe.$ gsutil hash -m $ASSET_PATH Hashes [base64] for $ASSET_PATH: Hash (md5): $FILE_HASH
1-2. Create a customer_set
object
With your CustomerSet asset file uploaded to Google Cloud Storage, you can create a customer_set
object using the asset URL for the CustomerSet asset file. You must specify either ADIDs for Android or IDFAs for iOS but not both.
The following example shows you how you can use your command-line interface to make an API request to generate a customer_set
object with the request body parameters you have specified as a .json
file. The parameters you have specified for your new customer_set
object are saved to the .json
file and delivered to the Campaign Management API as an HTTP POST request body. For more information about the parameters you can specify, see Create a new customer set.
$ cat customer_set.json
{
"title": "Test CustomerSet",
"description": "Test CustomerSet",
"id_type": "GOOGLE_ADID",
"data_file_path": "$CUSTOMER_SET_ASSET_URL"
}
$ curl -X POST \
-H "Authorization: Bearer $TOKEN" \
-H "Content-Type: application/json" \
-d "@customer_set.json" \
"https://api.moloco.cloud/cm/v1/customer-sets?ad_account_id=$AD_ACCOUNT_ID"
{
"customer_set": {
"id": "$CUSTOMER_SET_ID",
"title": "Test CustomerSet",
"description": "Test CustomerSet",
"id_type": "GOOGLE_ADID",
"status": "PREPARING",
"data_file_path": "$CUSTOMER_SET_ASSET_URL",
"created_at": "2020-07-01T15:34:43.323228Z",
"updated_at": "2020-07-01T15:34:43.323228Z"
}
}
As in the example, the response includes the CustomerSet ID, which is the value of the id
property in the customer_set
object. For more information about each of the parameters included in the response, see Create a new customer set.
Next steps
You can use the CustomerSet ID for your AudienceTarget entity. To learn how to create an audience_target
object, see Create a new audience target.
Updated about 1 month ago